
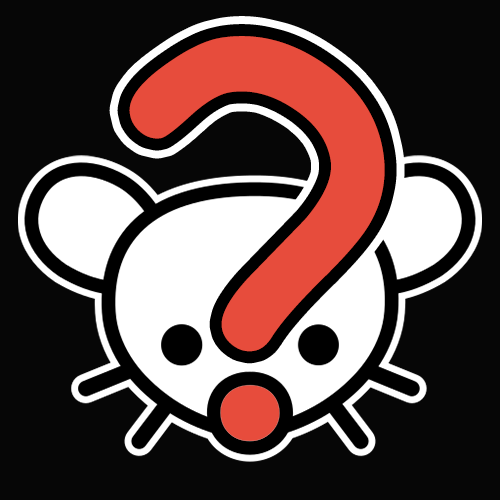
I Have No Meowth, and I Must Scream
I Have No Meowth, and I Must Scream
I couldn’t view this with Firefox or Gnome. ImageMagick to the rescue, though:
convert https://pub-be81109990da4727bc7cd35aa531e6b2.r2.dev/weofihweiof.jpg meme.jpg
They’d have to have a democracy in order for it to be called a democracy
After you hit a billion dollars, you should get a party and a nice trophy that says “You Won Capitalism!”, and then you should start from scratch again.
Just saw this community on the lemmy.world community list and it’s now a new favorite: !iso@lemmy.world
Wheel of Time on Amazon should’ve been animated. It might not have sold as well or whatever the execs wanted but it would’ve been way cheaper to produce something so much better.
I realize we’re probably not going to convince each other over some internet comments, but that’s not a philosophy I’d sign up for. Morality is subjective, and I’d rather choose moral principles that don’t involve me accepting being massacred.
It’s a nice thought, but doesn’t work out so well
Magnatiles are surprisingly fun
deleted by creator
I’m too lazy to convert that by hand, but here’s what chatgpt converted that to for SQL, for the sake of discussion:
SELECT
a.id,
a.artist_name -- or whatever the name column is in the 'artists' table
FROM artists a
JOIN albums al ON a.id = al.artist_id
JOIN nominations n ON al.id = n.album_id -- assuming nominations are for albums
WHERE al.release_date BETWEEN '1990-01-01' AND '1999-12-31'
AND n.award = 'MTV' -- assuming there's a column that specifies the award name
AND n.won = FALSE
GROUP BY a.id, a.artist_name -- or whatever the name column is in the 'artists' table
ORDER BY COUNT(DISTINCT n.id) DESC, a.artist_name -- ordering by the number of nominations, then by artist name
LIMIT 10;
I like Django’s ORM just fine, but that SQL isn’t too bad (it’s also slightly different than your version though, but works fine as an example). I also like PyPika sometimes for building queries when I’m not using Django or SQLAlchemy, and here’s that version:
q = (
Query
.from_(artists)
.join(albums).on(artists.id == albums.artist_id)
.join(nominations).on(albums.id == nominations.album_id)
.select(artists.id, artists.artist_name) # assuming the column is named artist_name
.where(albums.release_date.between('1990-01-01', '1999-12-31'))
.where(nominations.award == 'MTV')
.where(nominations.won == False)
.groupby(artists.id, artists.artist_name)
.orderby(fn.Count(nominations.id).desc(), artists.artist_name)
.limit(10)
)
I think PyPika answers your concerns about
What if one method wants the result of that but only wants the artists’ names, but another one wanted additional or other fields?
It’s just regular Python code, same as the Django ORM.
I’m pretty excited about PRQL. If anything has a shot at replacing SQL, it’s something like this (taken from their FAQ):
PRQL is open. It’s not designed for a specific database. PRQL will always be fully open-source. There will never be a commercial product.
There’s a long road ahead of it to get serious about replacing SQL. Many places won’t touch it until there’s an ANSI standard and all that. But something built with those goals in mind actually might just do it.
I don’t think you really have a choice TBH. Trying to do something like that sounds like a world of pain, and a bunch of unidiomatic code. If you can’t actually support 4 to 10 languages, maybe you should cut back on which ones you support?
One interesting thing you could try if you really don’t want to cut back is to try having using an LLM to take your officially supported code and transliterate it to other languages. I haven’t tried it at this scale yet, but LLMs are generally pretty good at tasks like that. I suspect that would work better than whatever templating approach you’ve used before.
If neither of those approaches works, everything speaks C FFI, and Rust is a modern language that would work well for presenting a C FFI that the other languages can use. You’re probably not hot on the idea of rewriting your Go tests into another language, but I think that’s your only real option then.
You might’ve seen this already, but here’s someone’s recreation of the Windows 98 theme in CSS: https://jdan.github.io/98.css/
One good part about having a kid is that you get to re-experience all of the fun kid stuff you remember, both as an adult and through the eyes of your kid. You can introduce your kid to your favorite shows/books/etc that you remember (and cringe at some of the stuff you forgot was in there).
deleted by creator
It’s probably not studied enough, but if you’re concerned about it, stick to gummies?
I wouldn’t call that an underlying moral code. You could call it laziness, I guess. I don’t want to live in a society where I have to worry “Will I get murdered today?”. If the entire state of Alabama starts getting murder happy it won’t affect me directly, but I’m sure going to start worrying that it’ll spread to other states where it does affect me.
I also would worry about the citizens of Alabama, don’t get me wrong. But that’s not from foundational philosophical reasoning, that’s just because of a human emotional response. If, for some inscrutable reason, Alabama collectively decided that they all loved murdering each other and only each other (excepting people unable to consent), I guess I’d just shrug and accept it? There’s some food for thought there, but it’s very similar to the willing cannibalism case.
Yes those are also examples of AI, see relevant Wikipedia article:
We need better terms to specify exactly what we mean, e.g. a numeric scale of intelligence or maybe even something more complex like a radar chart.